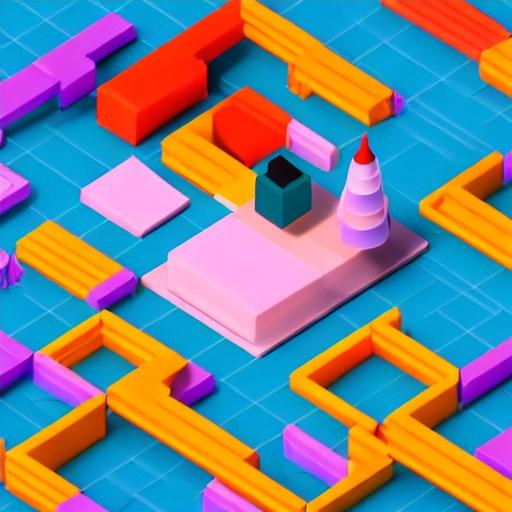
Lazy loading is a technique used in web development to improve website performance by loading content dynamically as it becomes visible to the user. Traditionally, when a web page loads, all the images, videos, and other media are loaded at the same time, even if they are not immediately visible. This can result in slower page load times and increased data usage.
To overcome this issue, lazy loading delays the loading of certain elements until they are needed or until they become visible on the user’s screen. This means that only the content that the user can see initially is loaded, while the rest is loaded as the user scrolls down the page. This can significantly improve page load times and reduce data usage, especially for websites with a lot of media content.
Implementing lazy loading on a website can be done using various techniques and libraries. One popular method is using JavaScript and the Intersection Observer API. The Intersection Observer API allows developers to track when an element enters or exits the viewport, which makes it perfect for lazy loading.
To implement lazy loading using the Intersection Observer API, you need to create a callback function that will be called whenever an element’s visibility changes. In this function, you can check if the element is visible and, if so, load the content dynamically. This can be done by updating the `src` attribute of an image or loading external content using AJAX.
Here’s a simplified example of how to implement lazy loading using the Intersection Observer API:
“`javascript
const observer = new IntersectionObserver(entries => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.setAttribute(‘src’, img.getAttribute(‘data-src’));
observer.unobserve(img);
}
});
});
const lazyImages = document.querySelectorAll(‘.lazy’);
lazyImages.forEach(image => {
observer.observe(image);
});
“`
In this example, the `IntersectionObserver` is created with a callback function that updates the `src` attribute of an image when it becomes visible. The `.lazy` class is added to the images that should be lazy loaded, and their actual source is stored in the `data-src` attribute.
By implementing lazy loading, you can greatly improve the user experience of your website. Users will see the initial content much faster, and unnecessary loading of hidden content will be avoided. This can lead to increased user engagement, better conversion rates, and improved search engine rankings.
In conclusion, lazy loading is a powerful technique for optimizing website performance by dynamically loading content as it becomes visible. By implementing lazy loading using the Intersection Observer API, you can significantly improve page load times and reduce data usage. So, if you want to enhance your website’s performance, consider implementing lazy loading for your media-rich content.