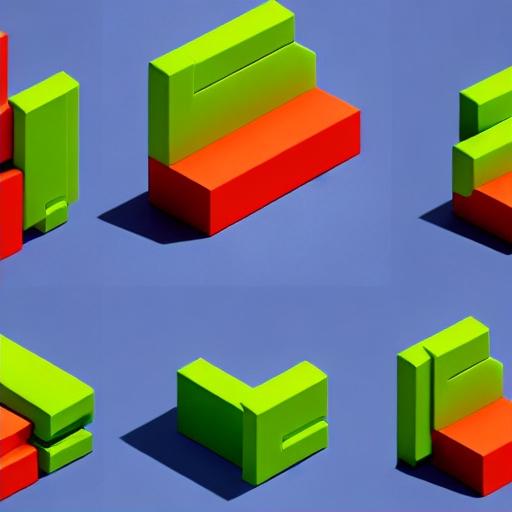
Lazy loading is a technique used in web development to optimize the loading time of a webpage by delaying the loading of certain elements until they are needed. This technique is especially useful for websites that contain large images or videos, as it allows the page to load quickly and improves the overall user experience.
To implement lazy loading, developers can use various frameworks and libraries. One popular library for lazy loading is the “intersection observer” API. This API allows developers to track when an element enters or exits the viewport of the user’s browser, triggering the loading of the element.
Here is an example of implementing lazy loading using the intersection observer API:
1. First, include the intersection observer polyfill if needed, as the API is not supported in all browsers by default.
“`html
“`
2. Next, add a placeholder element for the image or video that will be lazily loaded. This can be a simple
“`html
“`
3. Create a JavaScript function to handle the lazy loading. This function will use the intersection observer API to detect when the placeholder element enters the viewport.
“`javascript
function lazyLoadImage(entries, observer) {
entries.forEach(entry => {
if (entry.isIntersecting) {
const lazyImage = entry.target;
// Replace the placeholder with the actual image or video source
lazyImage.src = lazyImage.dataset.src;
// Stop observing the element once it has been loaded
observer.unobserve(lazyImage);
}
});
}
const options = {
rootMargin: ‘0px’,
threshold: 0.1
};
const observer = new IntersectionObserver(lazyLoadImage, options);
const lazyLoadImages = document.querySelectorAll(‘.lazy-load-image’);
lazyLoadImages.forEach(image => {
observer.observe(image);
});
“`
4. Finally, add the actual image or video source as a data attribute to the placeholder element. This can be done using the `data-src` attribute.
“`html
“`
By following these steps, lazy loading can be implemented on a webpage. As the user scrolls, only the elements that are visible will be loaded, reducing the initial load time of the page. This improves the website’s performance, especially on mobile devices with slower internet connections.